Module axi_lite
This document contains technical documentation for the axi_lite
module.
To browse the source code, visit the repository on GitHub.
This module contains a large set of AXI-Lite components written in VHDL. They are based around record types in axi_lite_pkg.vhd that make it convenient to work with the AXI-Lite signals.
See also the Module bfm for tools to efficiently simulate your AXI-Lite design.
axi_lite_cdc.vhd
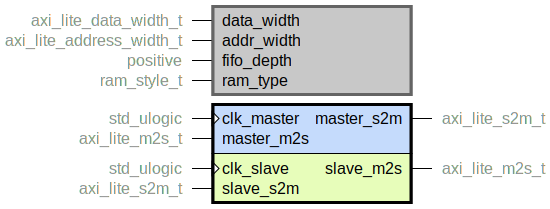
Clock domain crossing of a full AXI-Lite bus (read and write) using asynchronous FIFOs for the different channels. By setting the width generics, the bus is packed optimally so that no unnecessary resources are consumed.
Note
The constraints of asynchronous_fifo.vhd must be used.
Resource utilization
This entity has netlist builds set up with automatic size checkers in module_axi_lite.py. The following table lists the resource utilization for the entity, depending on generic configuration.
Generics |
Total LUTs |
FFs |
Maximum logic level |
---|---|---|---|
data_width = 32 addr_width = 24 |
199 |
290 |
4 |
axi_lite_mux.vhd
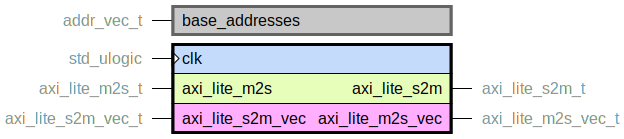
AXI-Lite mux, aka simple 1-to-N crossbar.
The base_addresses
generic is a list of base addresses for the N slaves.
If the address requested by the master does not match any base address, this entity
will send AXI decode error DECERR
on the response channel (RRESP
or BRESP
).
There will still be proper AXI handshaking done, so the master will not be stalled.
Warning
This entity is written to be used in a register bus, and is designed for simplicity and low
resource utilization.
An assumption is made that the AXI-Lite master does not queue up reads or writes.
I.e. after an AR
transaction, it does not send another ARVALID
before the
R
transaction has happened.
Same for AW
and W
/B
.
If the AXI-Lite does queue up transactions it can lead to locking the bus.
However, if you are using this entity then you probably have a axi_to_axi_lite.vhd instance upstream. This entity will by design not queue up transactions.
Resource utilization
This entity has netlist builds set up with automatic size checkers in module_axi_lite.py. The following table lists the resource utilization for the entity, depending on generic configuration.
Generics |
Total LUTs |
FFs |
Maximum logic level |
---|---|---|---|
(Using wrapper axi_lite_mux_netlist_build_wrapper.vhd) |
516 |
28 |
5 |
axi_lite_pipeline.vhd
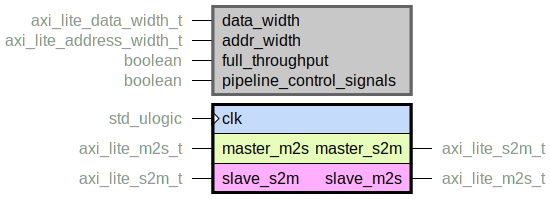
Pipelining of a full AXI-Lite bus (read and write), with the goal of improving timing on the data and/or control signals.
The default settings will result in full skid-aside buffers, which pipeline both the data and control signals. However the generics to handshake_pipeline.vhd can be modified to get a simpler implementation that results in lower resource utilization.
axi_lite_pkg.vhd
Data types for working with AXI4-Lite interfaces. Based on the document “ARM IHI 0022E (ID022613): AMBA AXI and ACE Protocol Specification” Available here: http://infocenter.arm.com/help/index.jsp?topic=/com.arm.doc.ihi0022e/
axi_lite_simple_read_crossbar.vhd

Simple N-to-1 crossbar for connecting multiple AXI-Lite read masters to one port. This is a wrapper around axi_simple_read_crossbar.vhd. See that entity for details.
axi_lite_simple_write_crossbar.vhd

Simple N-to-1 crossbar for connecting multiple AXI-Lite write masters to one port. This is a wrapper around axi_simple_write_crossbar.vhd. See that entity for details.
axi_lite_to_vec.vhd
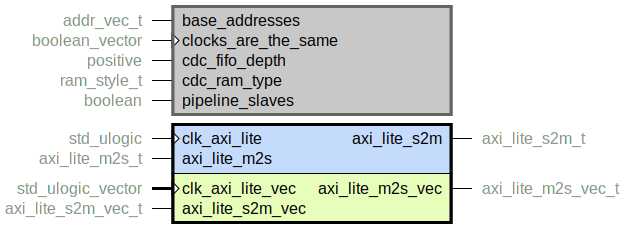
Convenience wrapper for splitting and CDC’ing a register bus based on generics. The goal is to split a register bus, and have each resulting AXI-Lite bus in the same clock domain as the module that uses the registers. Typically used in chip top levels.
Instantiates axi_lite_mux.vhd and axi_lite_cdc.vhd.
axi_to_axi_lite.vhd
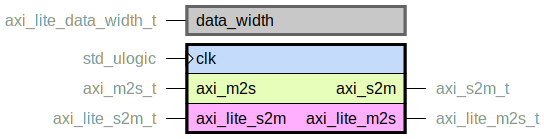
Convert AXI transfers to AXI-Lite transfers.
This module does not handle conversion of non-well behaved AXI transfers.
Burst length has to be one and size must be the width of the bus. If these
conditions are not met, the read/write response will signal SLVERR
.
This module will throttle the AXI bus so that there is never more that one outstanding transaction (read and write separate). While the AXI-Lite standard does allow for outstanding bursts, some Xilinx cores (namely the PCIe DMA bridge) do not play well with it.
axi_to_axi_lite_vec.vhd
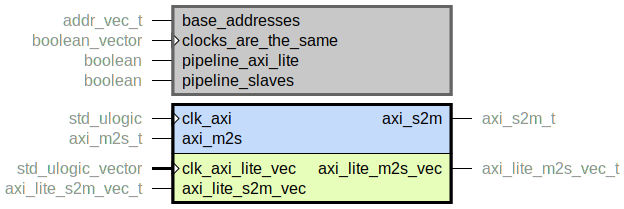
Convenience wrapper for converting a AXI bus to AXI-Lite, and then splitting and CDC’ing a register bus. The goal is to split a register bus, and have each resulting AXI-Lite bus in the same clock domain as the module that uses the registers. Typically used in chip top levels.
Instantiates axi_to_axi_lite.vhd, axi_lite_mux.vhd and axi_lite_cdc.vhd.